Python basics 2: What's your name?
In "Python Basics 2: What's Your Name?", Daniël Roos introduces the foundational elements of Python programming, focusing on variables, data types, and basic operations. This lesson guides beginners through creating a simple program that interacts with the use.
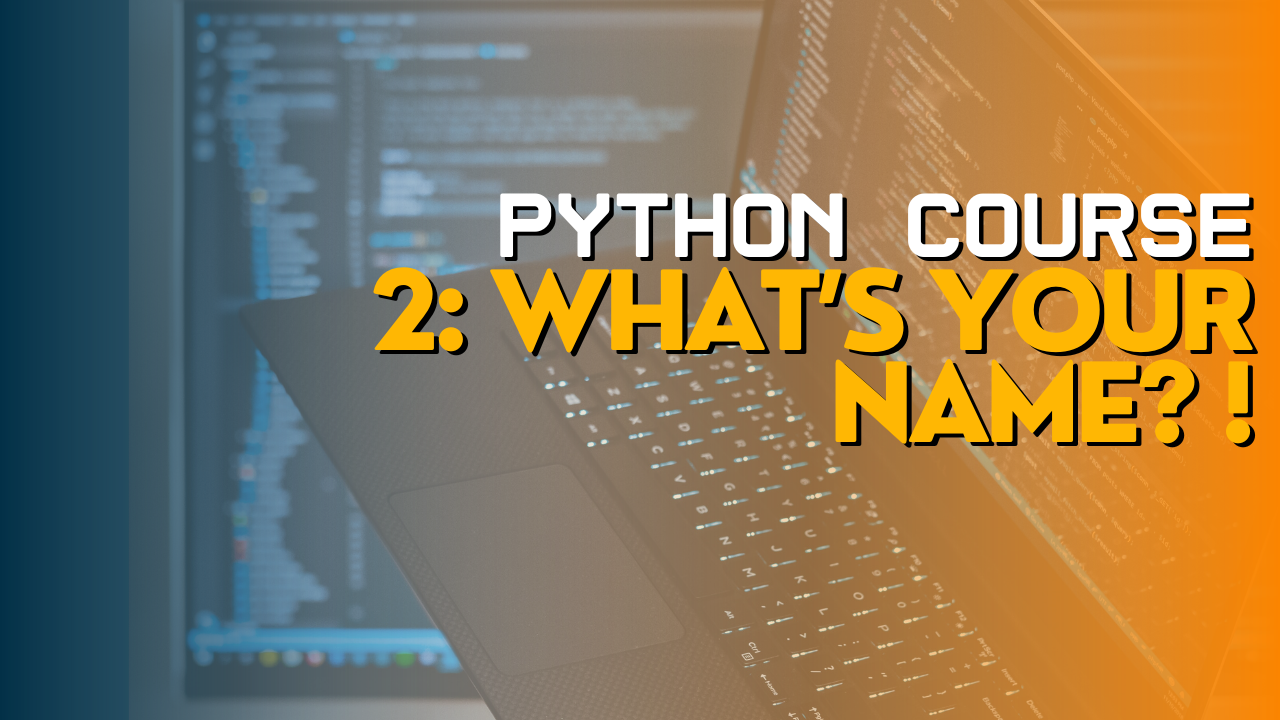
Welcome back to this introduction course on Python. According to the 2023 Stack Overflow Developer survey, the third most used programming language in the world.
In this second lesson, you'll learn about the basic building blocks of a good Python program. Variables, data types, and basic operations. We'll finish this lesson by creating a small program that asks you questions and uses the answers later.
Variables
Programming languages, such as Python, usually input and output data. In between, the program does certain actions. These actions are functions, and the data we use in them often gets stored in variables. think of them as labeled boxes with a certain value stored inside. Just like you'd put food in the fridge to preserve and eat later.
We'll create a simple variable that stores our name. What would you call a box in real life, when storing a name in it? Name right. We'll do the same thing. Start by creating a new Python file for this lesson. Right-click below "lesson1.py" to create a new file named "lesson2.py".
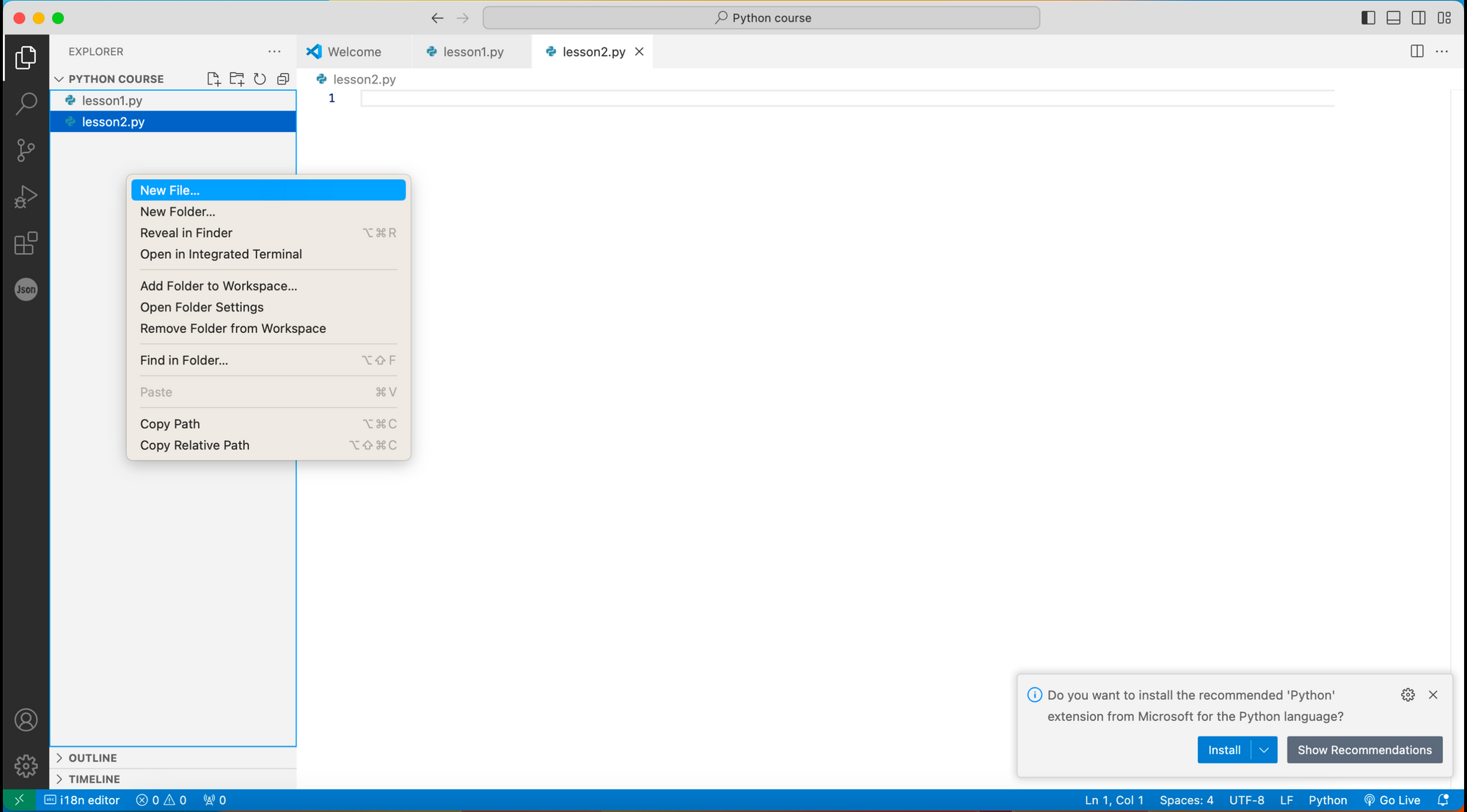
Now open this new file and type in the following code:
name = 'Insert your name'
This creates a new variable that stores the name you filled in between the quotation marks. I'll explain why we need them later.
With just a variable storing our name, we can't do anything. Let's use the print function from the previous lesson to show our variable. Type:
print (name)
Your script should now look like this:
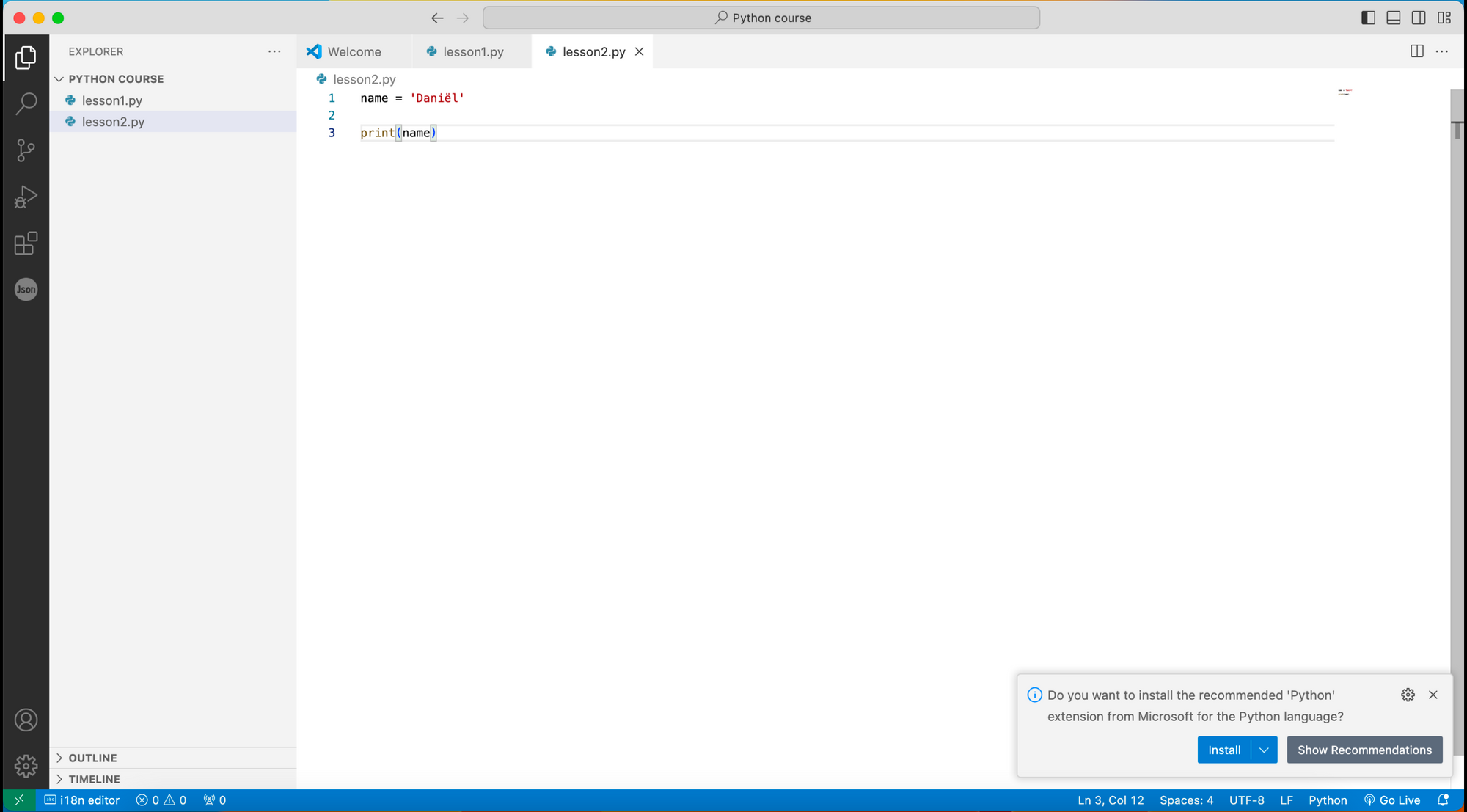
Let's run it to check if it works. Right-click on the file name in the left pane and click "Open in integrated terminal":
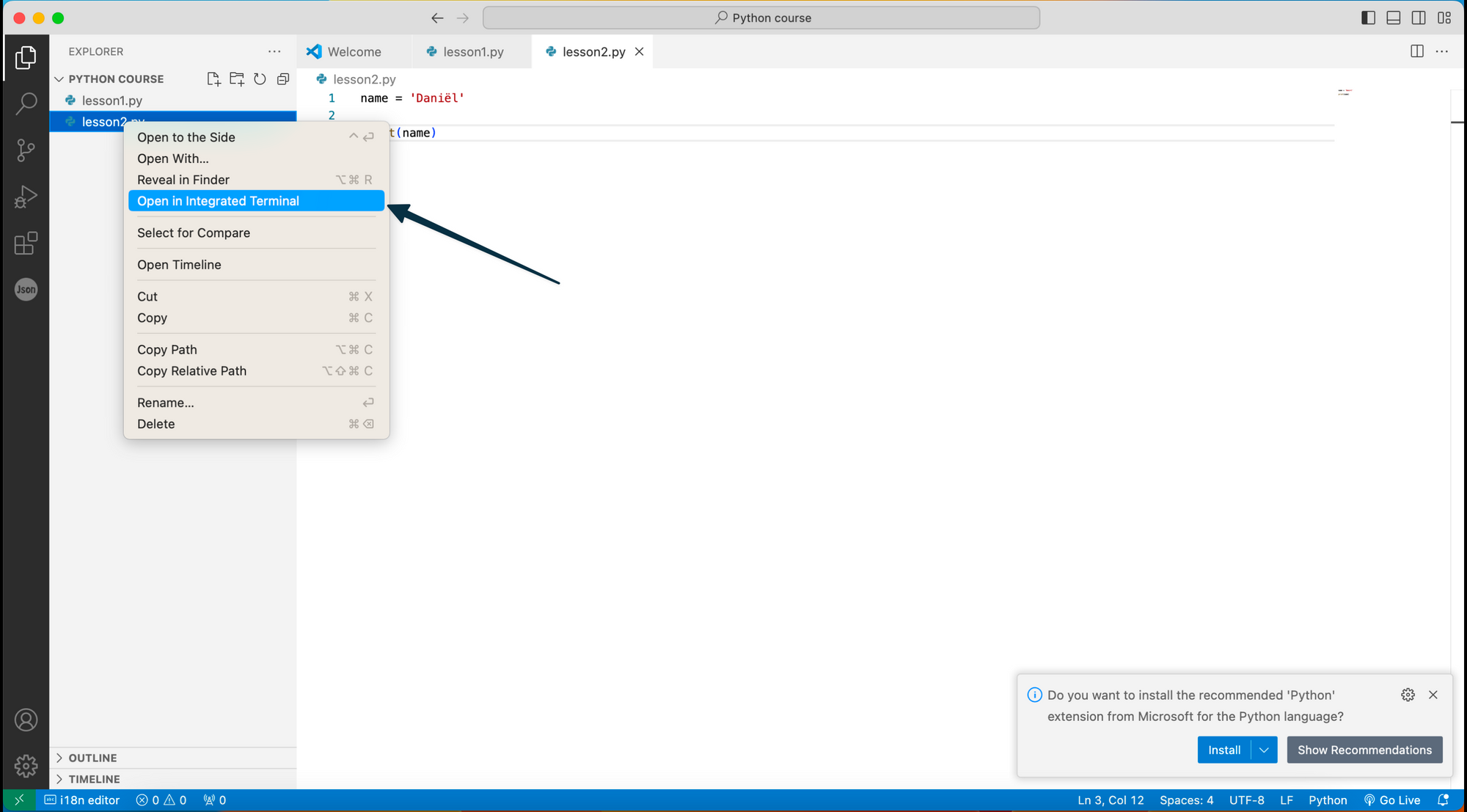
This will open a new terminal window in Visual Studio Code, already active in the directory of your files. Cool right?
Go ahead and type:
python 3 lesson2.py
You should now see the input of your variable pop up:
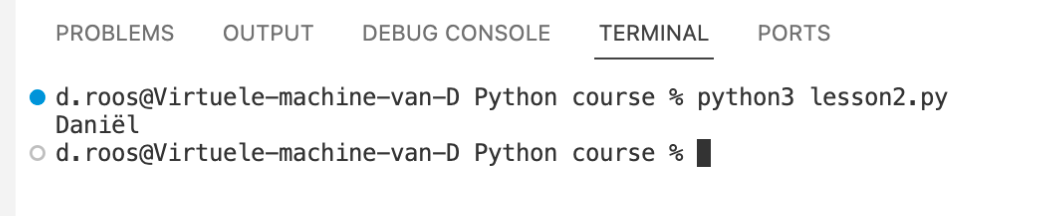
Data types
One of the most used, but in my opinion also most boring things to learn in Python are the different data types. To avoid overcomplicating things, I'll start by introducing only the 4 datatypes that I use most. These are:
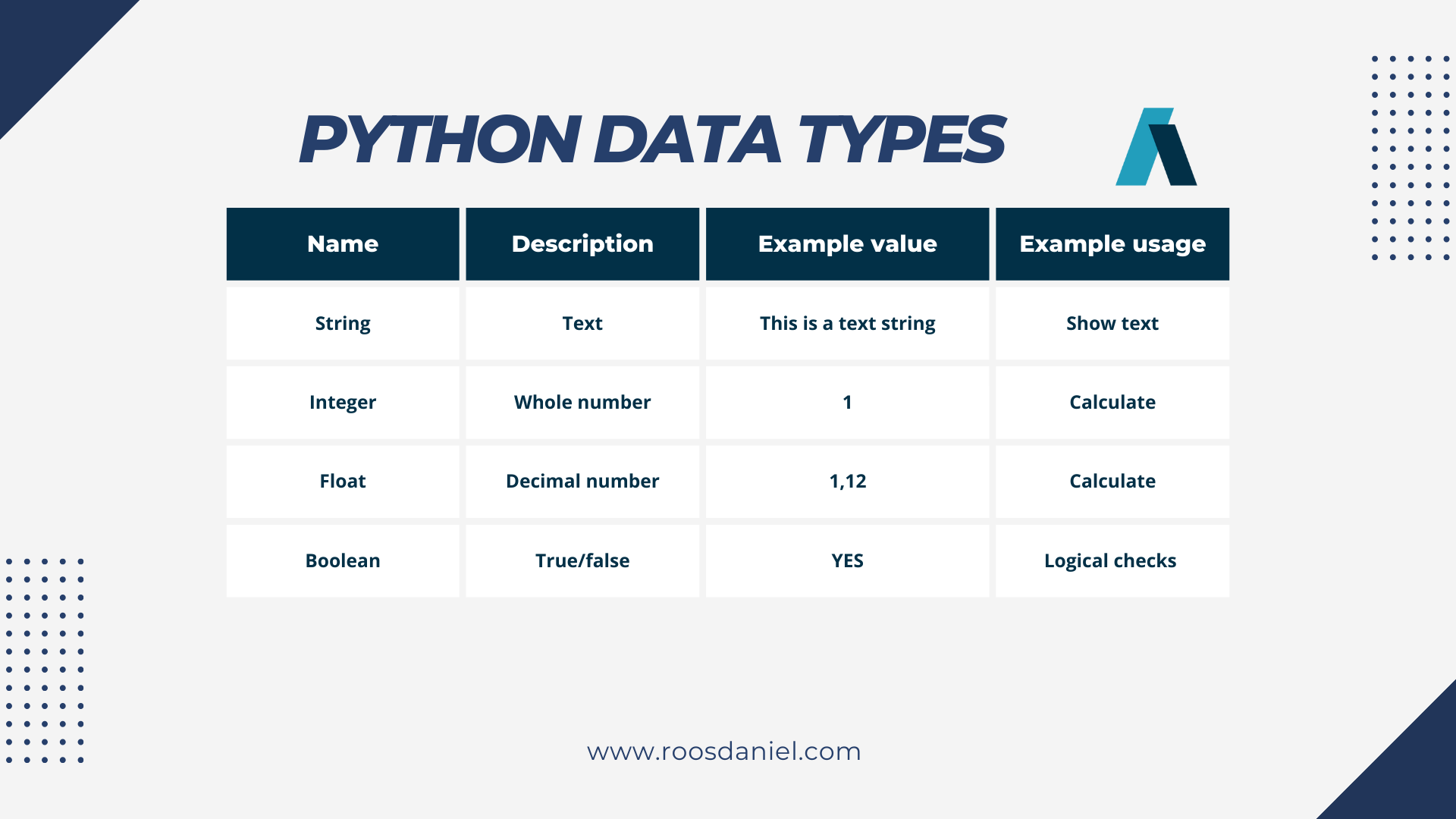
Luckily, Python automatically determines the data type, making it dynamically typed. You can always check the data type Python assigned to something by using the type() function. Let's use this by typing the following line below the print line in your script:
print(type(name))
Then run the code, the return should include 'str', which stands for string. That's correct since names are text values.
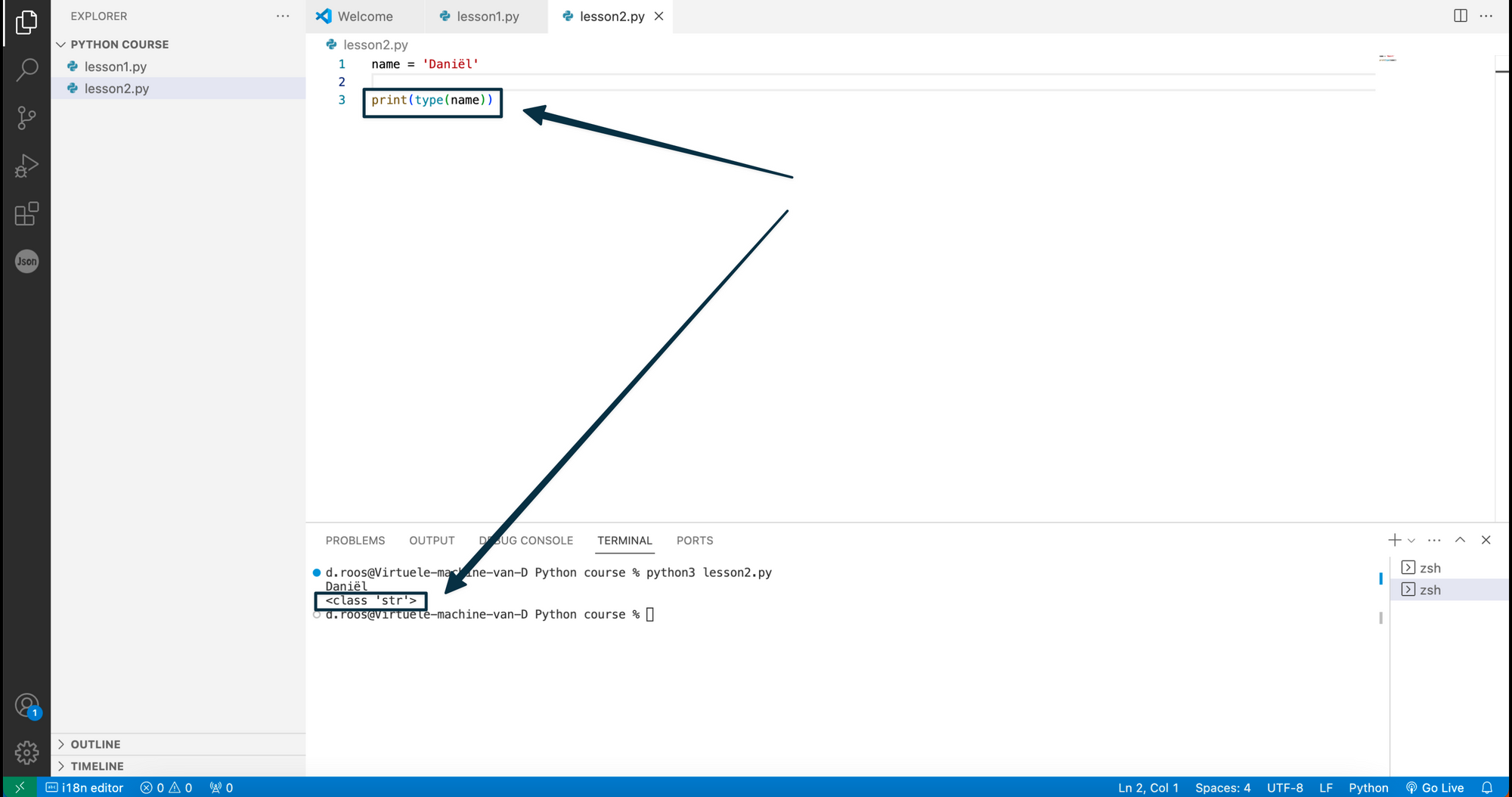
Basic operations
Now we've got to know variables and data types, let's use them in some simple calculations. Python supports a lot of them:
Mathematical operations: Addition, subtraction, multiplication, and more.
String operations: Concatenation, repetition, and slicing.
Comparison Operations: Comparing values to get a Boolean result.
Let's try one of each operation category specified above. Make 3 new lines:
print(4*4)
print('Hello' + ' ' + 'World')
print(5>8)
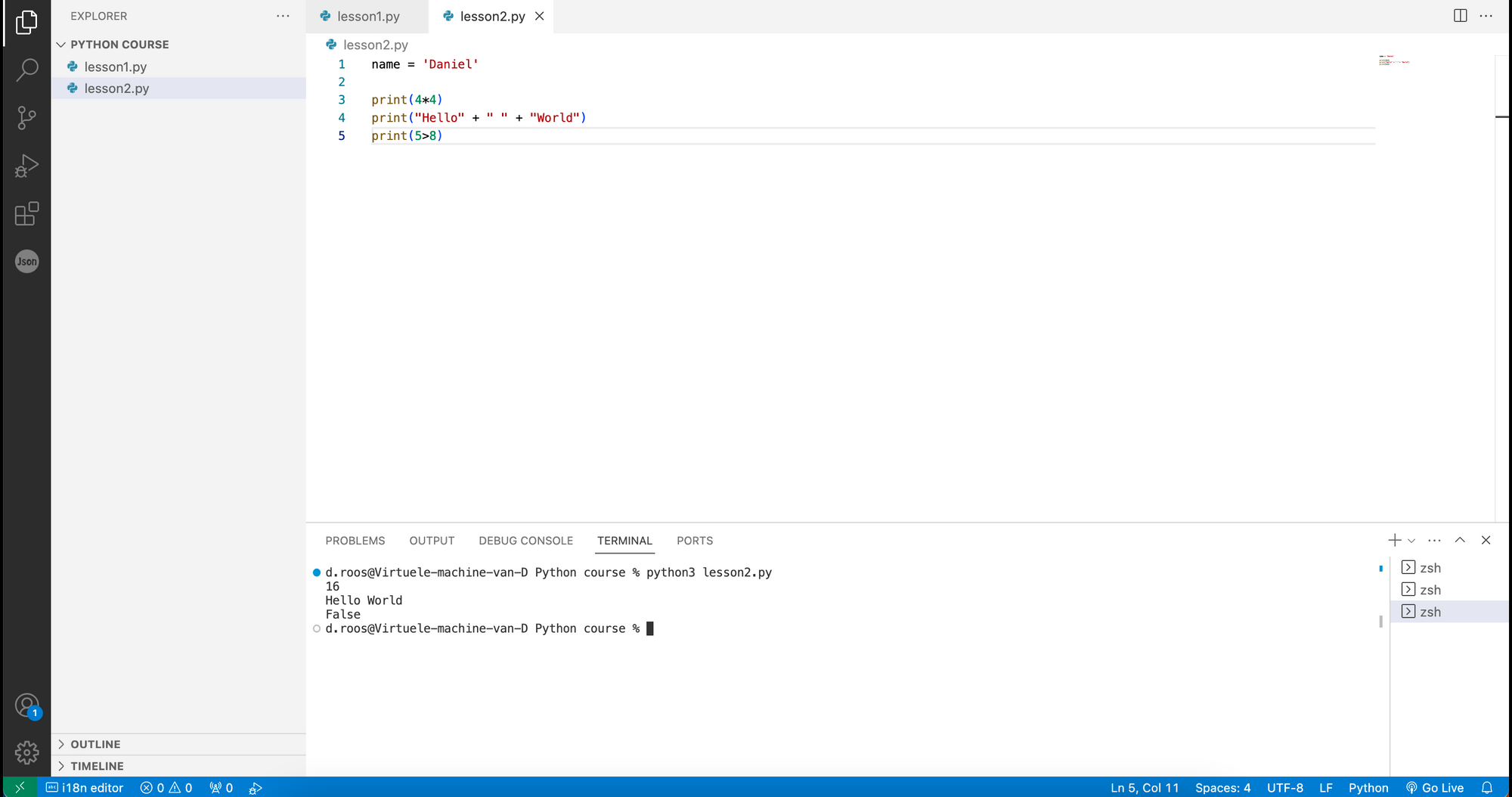
First we multiply two numbers, then we concatenate text, and lastly, we print the outcome of a comparison. It should be false in this case, since 5 is smaller than 8.
By now, you may have noticed we put text (strings) between " and ' signs. This is used to tell python it needs to treat our input as text, not a function or something else. Both " and ' have the same effect and can be used together when trying to print a sentence which has " already, like this:
print('He said, "GO"')
Receiving user input
Python can also retrieve user input. This allows you to ask question and store the answers, for example. Look at the following code:
user_name = input("Enter your name: ")
print(f"Hello, {user_name}!")
Try to understand what this piece of code does.
First we create a new variable named user_name. We then assign "input("Enter your name: "to it. Try and run only this line. When asked, insert your line and press enter.
To do something with the variable we created, let's print it in a customized message. For this, we want to include a variable in our print statement. We need to tell Python to do this properly. We don't want it to print our variable name instead of its contents. This is where the letter 'f' comes into play. It tells Python that we're going to print what's called an 'f-string'. F-strings allow us to embed variables within strings. We then only need to tell Python where within our string we want to embed the variable by inserting its name between curly brackets {}.
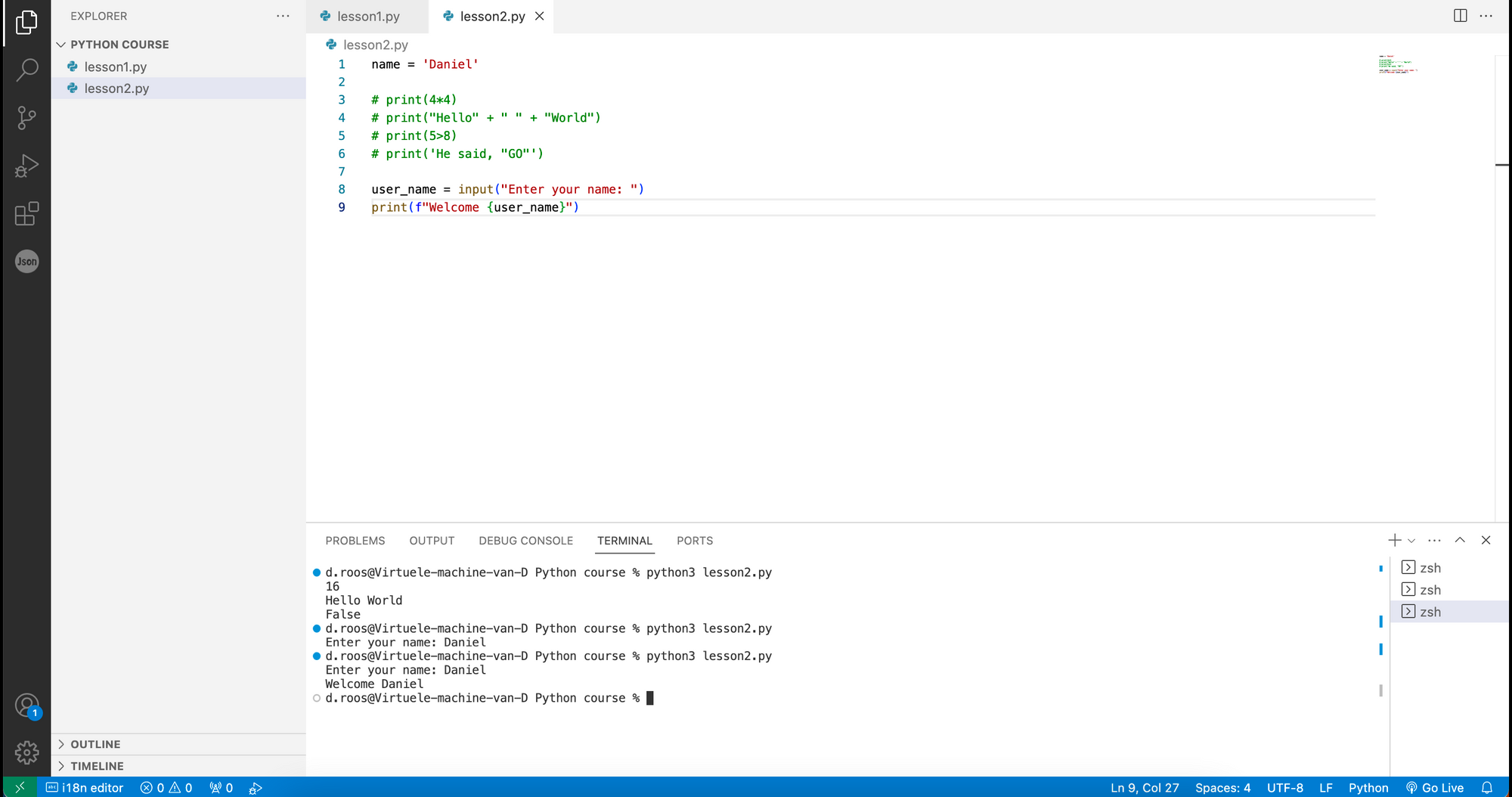