Python basics 3: Letting code make decisions
Explore the fundamentals of Python with "Python Basics 3: Letting Code Make Decisions," where Daniël Roos demystifies conditional logic and control flow using intuitive examples. This concise, beginner-friendly post is an essential read for anyone starting their journey in Python programming.
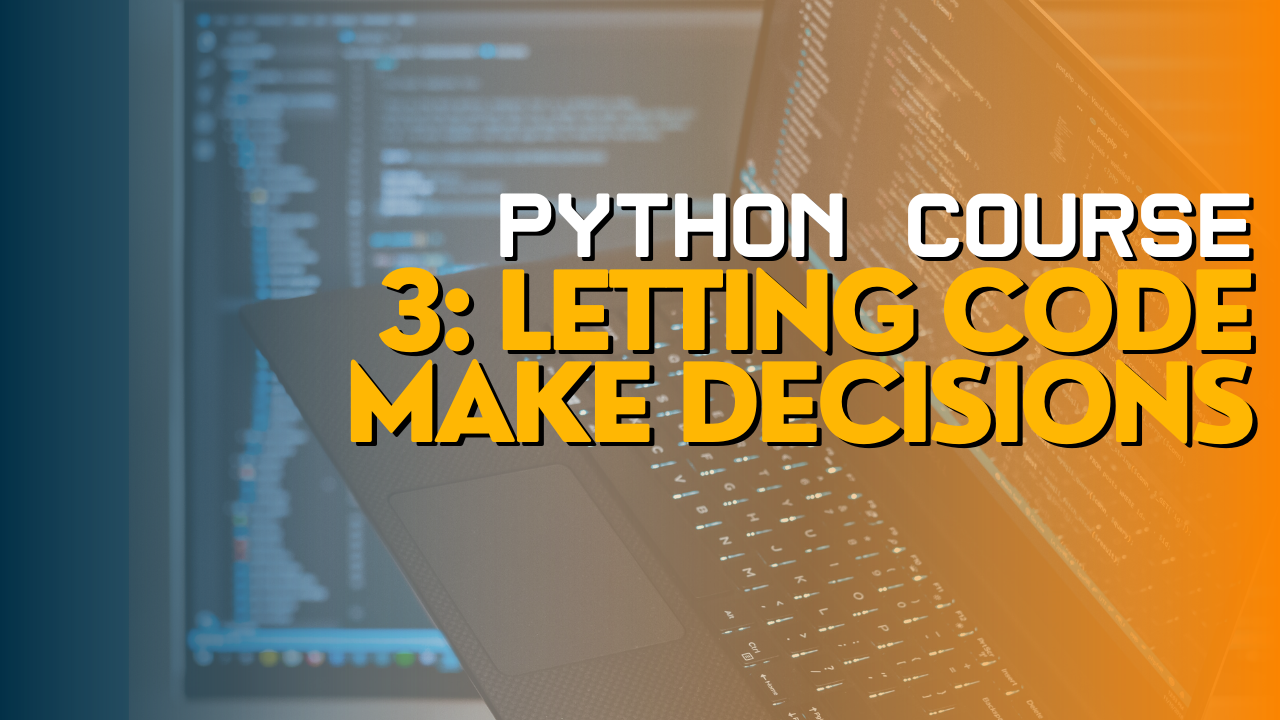
Welcome back to this introductory course on Python. According to the 2023 Stack Overflow Developer survey, the third most used programming language in the world.
This article covers an exciting topic, the use of so-called conditional logic to let your script make decisions.
Introduction to conditional logic and control flow
Conditional logic in combination with a concept called control flow are like the traffic lights you come across while driving on the streets. They tell you whether you're allowed to continue driving or not. Based on the signals of other traffic light.
During the last session we look at comparison operators, such as 5 > 2, to check whether 5 is bigger than 2. Try to recreate this script in a new file for this lesson and notice the output:
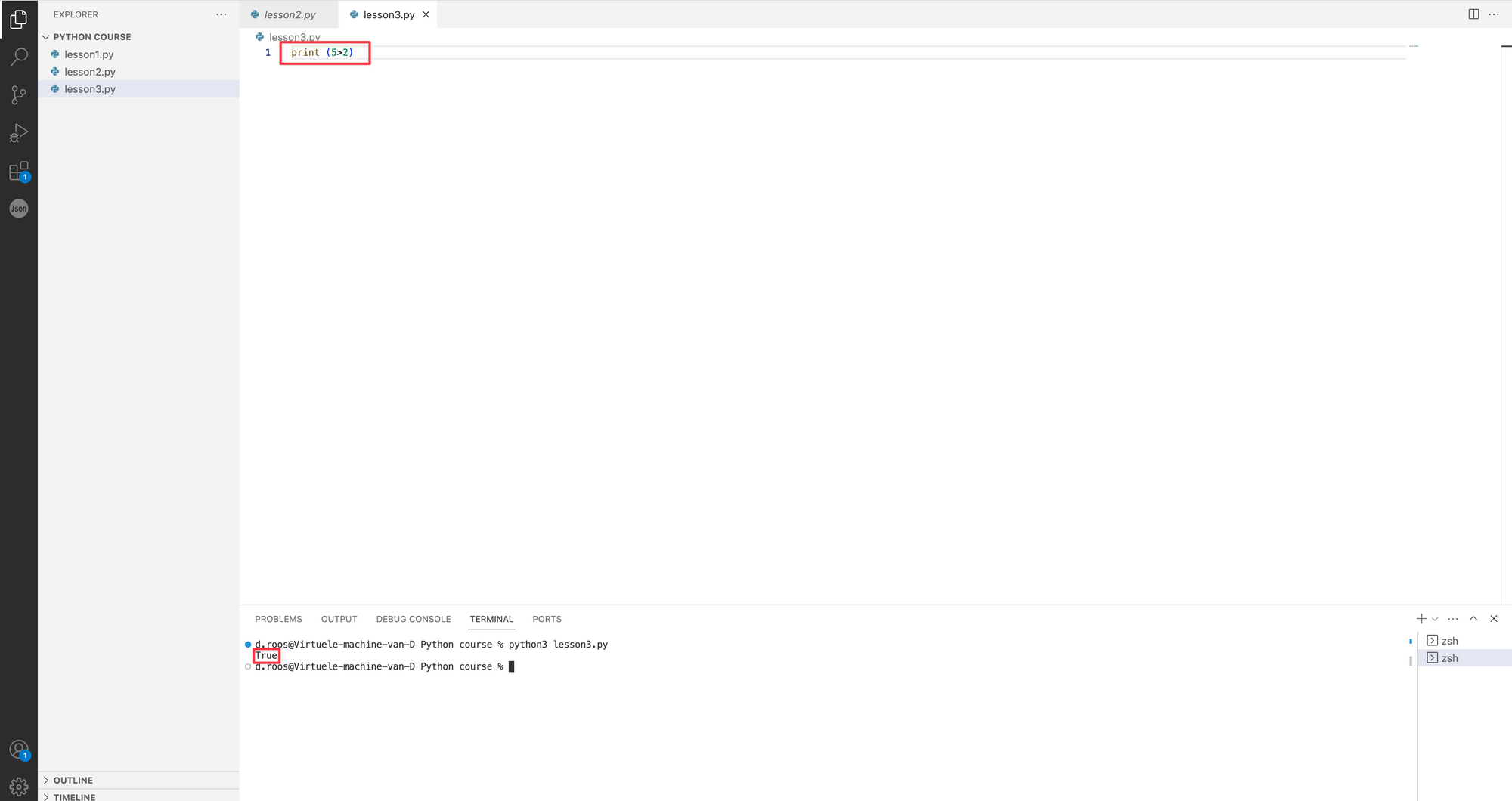
As you can see, this comparison operation returned "true". If we reverse the numbers to 2 > 5, it returns "false".
Try to think of these two boolean values (true and false) as red and green traffic lights.
We want to use these comparisons in our code to tell the program to do something based on the signal (true or false).
Let's think of a real-life use case. You're working on a big project and must check whether your data is clean. You have a specific financial column that should always contain data. You want to make sure it's not empty before doing calculations on it. this translates into the following logic:
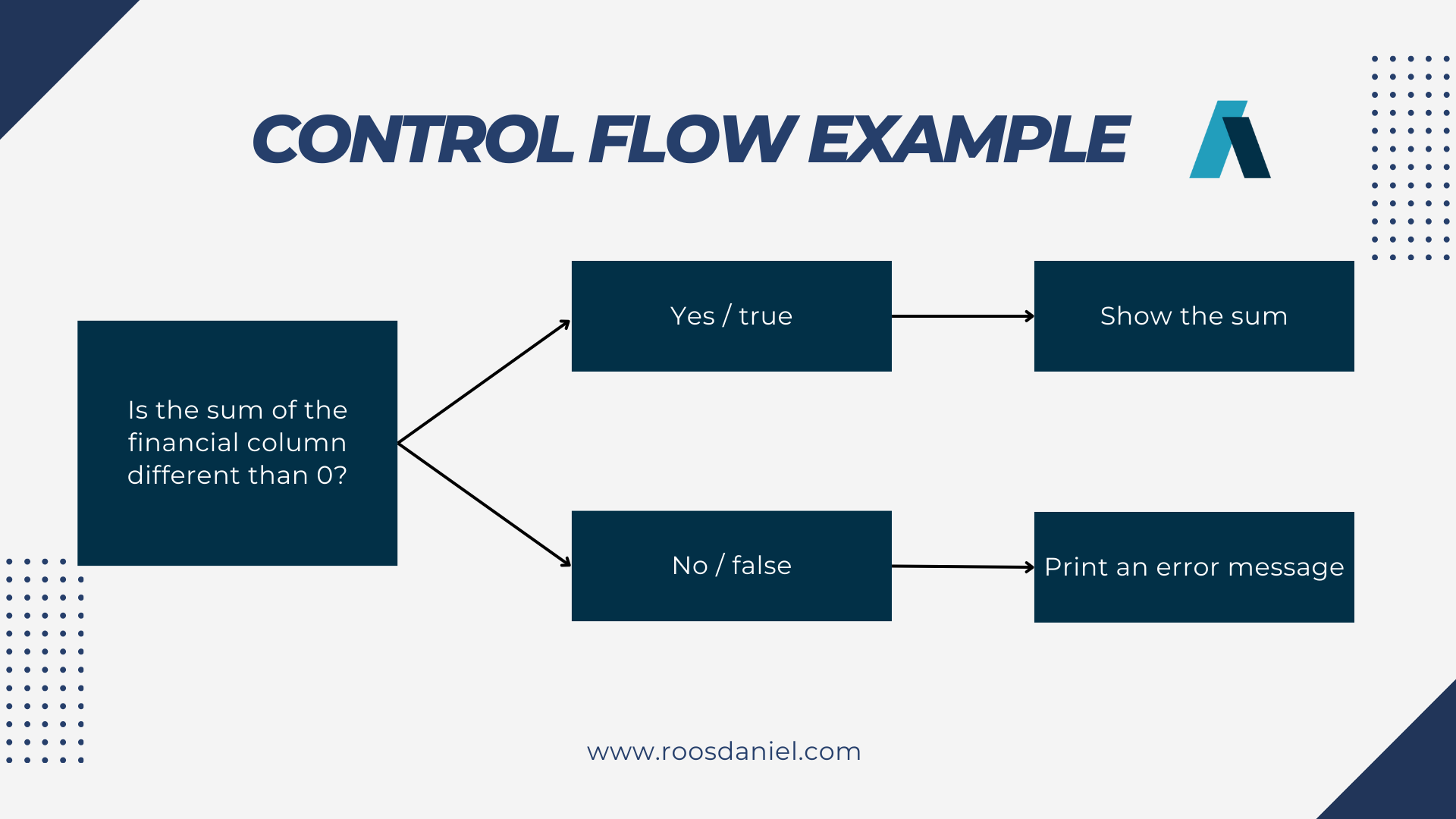
A good way to translate this into Python code would be by using an if statement in combination with a sum on the entire column. To do this, we need to comment out the existing logical check and add four lines to our Python file.
import pandas as pd
# Sample data: list of dictionaries
data = [
{'Product': 'Product A', 'Revenue': 1000},
{'Product': 'Product B', 'Revenue': 1500},
{'Product': 'Product C', 'Revenue': 2000},
{'Product': 'Product D', 'Revenue': 2500},
{'Product': 'Product E', 'Revenue': 3000},
{'Product': 'Product F', 'Revenue': 3500},
{'Product': 'Product G', 'Revenue': 4000},
{'Product': 'Product H', 'Revenue': 4500},
{'Product': 'Product I', 'Revenue': 5000},
{'Product': 'Product J', 'Revenue': 5500}
]
# Create DataFrame
df = pd.DataFrame(data)
# Sum the 'Revenue' column
print(df['Revenue'].sum())
Don't worry when you don't understand the lines completely. I will explain these steps in another lesson. For now, it's enough to understand that the print statements print the sum of the values in the second line. When importing an Excel sheet, these could be our column values.
Next, open the terminal and say pip3 install pandas. This installs a so-called library containing functions to deal with Excel sheets.
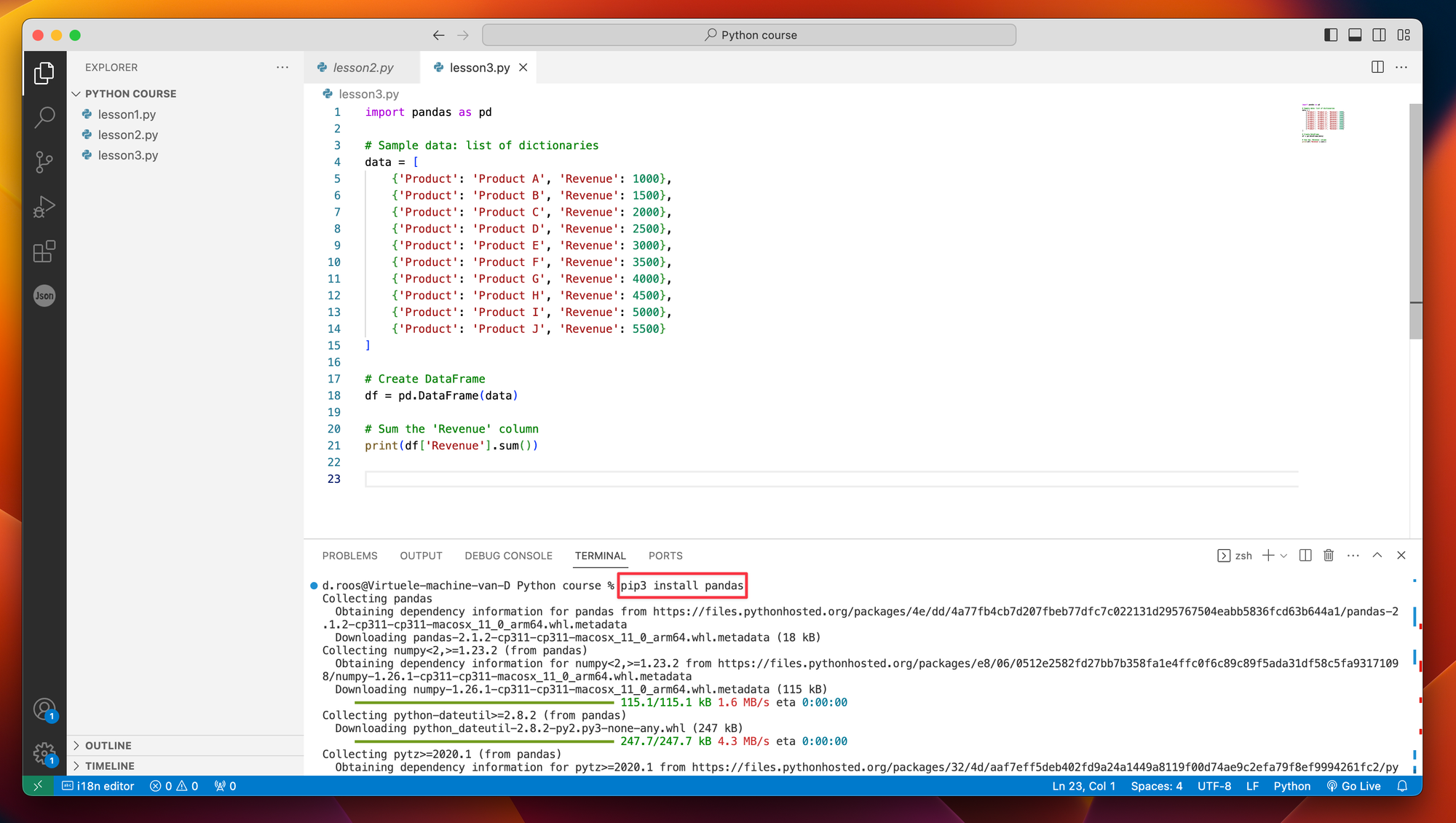
Then run the code:
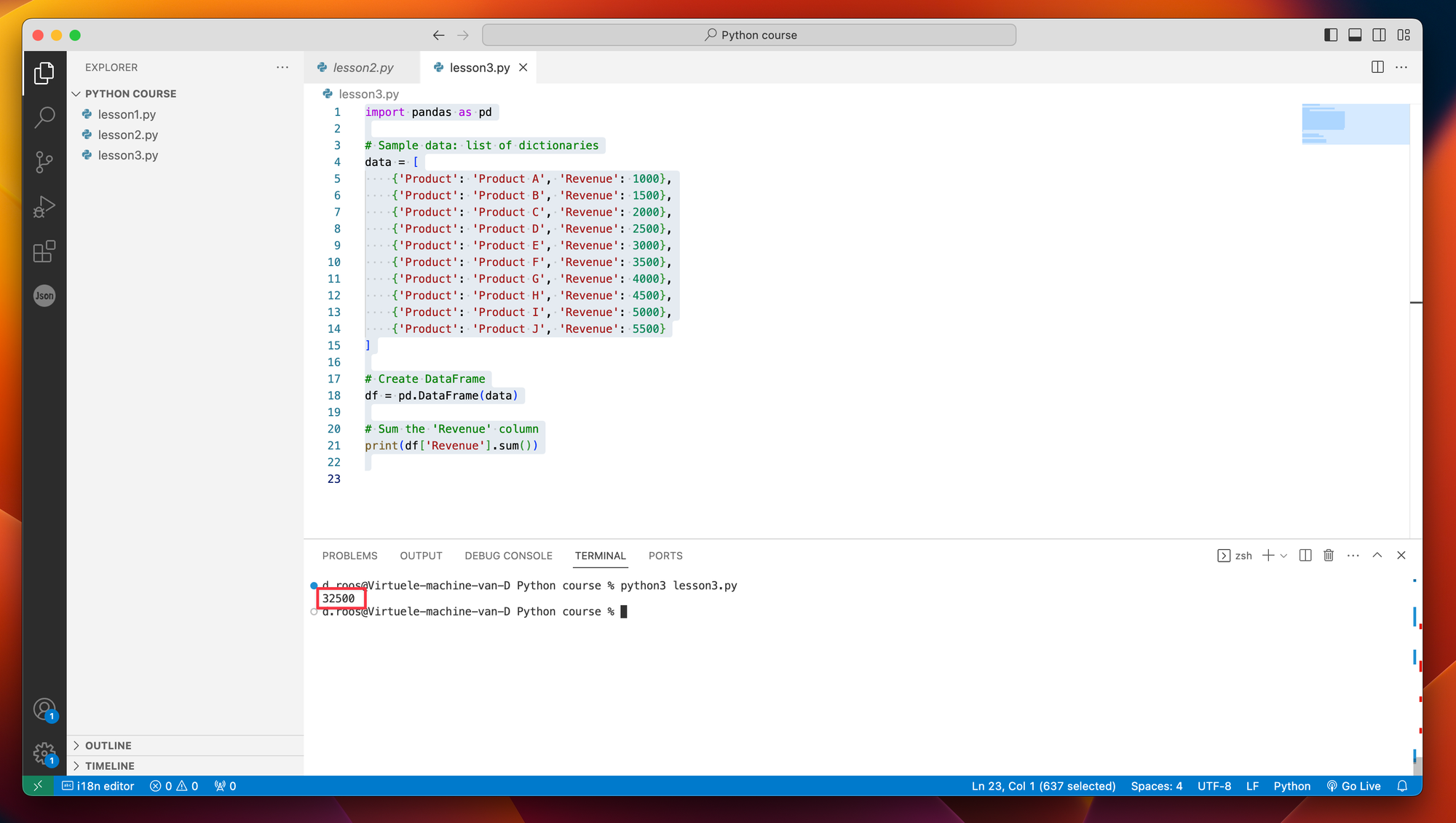
Now, let's apply the conditional logic we discussed. We want to check if the total revenue is different than 0. If it's true, we'll print a message saying the revenue is sufficient. If not, we'll print a message saying the revenue is too low. Here's how you can do it:
- Store the sum of the revenue column in a variable named: total_revenue
- Create an if statement comparing the sum of the total revenue to 0. If it is not 0, print a formatted string: "Total revenue {total_revenue} is sufficient."
- If the sum is equal to 0, print "Total revenue is insufficient"
Try to look at this example and then type out the code yourself:
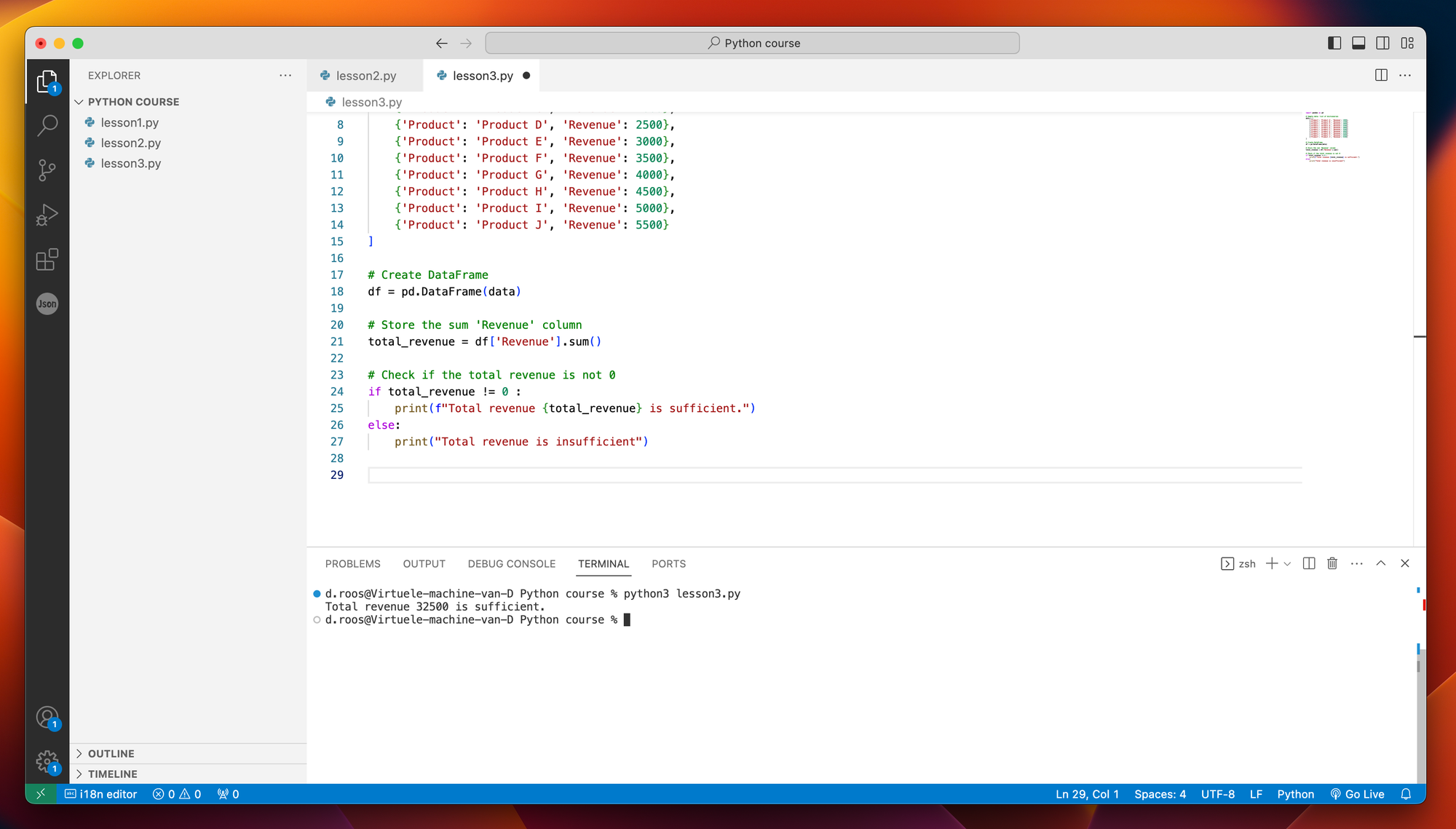
Did your code return the right string? If so, congratulations on completing this exercise! You've now taken your first steps in understanding and applying conditional logic and control flow in Python, which are essential skills in your programming journey.